Basics of Linux/Unix Shell Scripting
Well developing a script in Linux or Unix can be extremely easy or surprisingly complex and it all depends on how much you are familiar with Unix/Linux commands and trying to get the script to do. In this blogpost we'll see and learn "How to create shell script on a Linux/Unix machine" but before doing so lets get understand terms like - Terminal, Shell, and Kernel.
What is a Terminal ?
A terminal emulator is a program that allows the use of the terminal in a graphical environment. As most people use an OS with a graphical user interface (GUI) for their day-to-day computer needs, the use of a terminal emulator is a necessity for most Linux server users. The main purpose of a terminal is not to process information (like a typical computer) but to send commands to another system. So in brief we can say that the terminal is a program that provides the user with a simple command-line interface and performs the following 2 tasks:
Takes input from the user in the form of commands
Displays output on the screen
Also we can refer the terminal as a dumb thing coz it does not know what to do with the input, so it needs another program to process it, and in most cases, it’s the Shell.
Unix/Linux Operating System is made of many components, but its two main components are : Kernel and Shell.
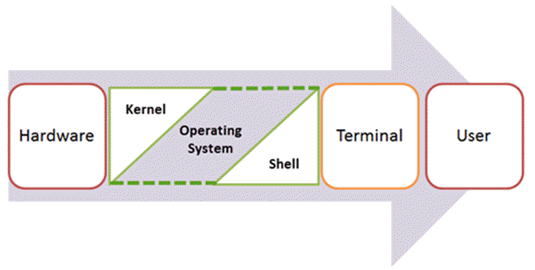
What is a Kernel ?
A Kernel is at the nucleus of a computer. It makes the communication between the hardware and software possible. While the Kernel is the innermost part of an operating system, a shell is the outermost one. As soon as the shell converts the user’s command into kernel-understandable form, the kernel is responsible to execute the commands with the help of its 2 components which are OS libraries and Device Drivers interacting with Application software and device hardware respectively.
What is a Shell ?
After writing our commands on the terminal, when we press the Enter key, the terminal passes those commands to another program to figure out what the user wants to do, and in most cases, that program is the Shell, which forms the outer layer of Linux OS, which performs the following functions:
Interprets the command given by the end-user
Checks the syntax of the command and then Checks whether the command is correctly used or not.
If everything is correct, the shell converts the command Into a kernel-understandable form and passes it to the kernel. Else, It returns an error message.
The $ prompt appears on the terminal waiting for the next command, Irrespective of whether the previous commands were correct or not.
So basically the shell is a mediator between the end-user and an operating system service which provides users with an interface and accepts human-readable commands into the system and executes those commands which can run automatically and give the program’s output in a shell script.
Identifying the shell
There are several shells that are widely used, such as Bourne shell (sh) and C shell (csh). Each shell has its own feature set and intricacies, regarding how commands are interpreted, but they all feature input and output redirection, variables, and condition-testing, among other things. Now lets see below commands to determine what kind of shells are available or your are using: 1) Use this command to determine which shell you are using:
$ echo $SHELL
/bin/bash

2) To determine your primary shell, execute below command:
$ grep $USER /etc/passwd
imjhachandan:x:1000:1000:Jha Chandan,,,:/home/imjhachandan:/bin/bash

3) Check the /etc/shells file to determine what shells are available on a Linux system:
$ more /etc/shells
# /etc/shells: valid login shells
/bin/sh
/bin/bash
/usr/bin/bash
/bin/rbash
/usr/bin/rbash
/bin/dash
/usr/bin/dash
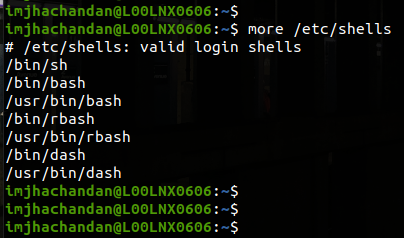
Which shell is best for any individual depends largely on what they’re used to using since much of the syntax in scripting does not represent the commands that you will find in /bin, /usr/bin or /usr/local/bin. Instead, they are part of the shell itself and are called “built-ins”. This includes the commands used for looping (e.g., for and while). One easy choice for scripting is to use whatever shell you use on the command line since, after all, you’ll be more or less comfortable using it.
Selecting your shell
To determine which of the available shells will run the commands in your script, make the first line of your script look like one of these:
/bin/sh
/bin/bash
/usr/bin/bash
/bin/rbash
/usr/bin/rbash
/bin/dash
/usr/bin/dash
When the first line of your script identifies the shell to be used, that shell will run the commands in the script. If you do not include the shell as the first line in a script, the shell you use when you invoke the
script will be the one that runs it.
What is Shell Scripting?
Shell Scripting is an open-source computer program designed to be run by the Unix/Linux shell. It is a program to write a series of commands for the shell to execute. It can combine lengthy and repetitive sequences of commands into a single and simple script that can be stored and executed anytime which, reduces programming efforts.
Creating and Running a script
Any command you run on the Linux command line can be run in a script provided it’s compatible with the specified shell. Use your favorite text editors (like vi, vim, nano, gedit etc..c ) and type in your commands. Here’s a very simple script named as "today" that shows the current date in a day-month-year format.
$ vi today
$ cat today
#!/bin/bash
date +"%d-%m-%Y"
Execute today script as show below
$ sh ./today
29-08-2021
OR
$ . today
29-08-2021
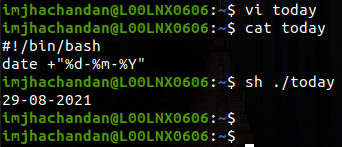
Adding comments in a script
It’s a good idea to add comments to scripts that explain what a script is meant to accomplish- especially when a script is long or the syntax is complicated. Just start the comment with a # sign. Comments can be lines by themselves or added to the end of commands in the script.
For example, see this DateTime script:
#!/bin/bash
# Script Name : DateTime
# Script Info: Display the date/time repeatedly in a loop
# Created by/On : ImJhaChandan/29-08-2021
while true
do
echo -n Now Time is...
date
sleep 5s # sleep 5 Seconds
done
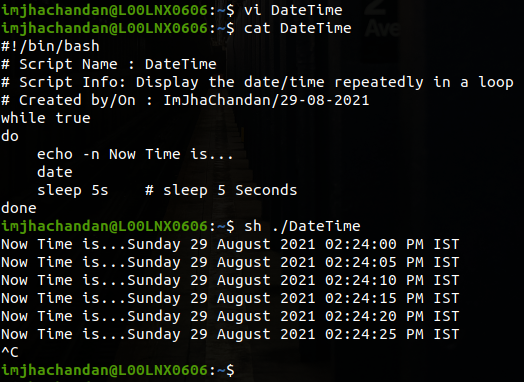
Making the script file executable
To make a script executable, use the chmod command and make sure that the intended users can run it. For example:
$ chmod 700 SampleScript # you can run it
$ chmod 755 SampleScript # everyone can run it
If a script isn’t set up to be executable, it can still be run using the "sh ./" OR “.” built-in that “sources” (i.e., reads and runs) the script.
$ sh ./today
29-08-2021
OR
$ . today
29-08-2021
Using an if command in a script
The if command allows you to test conditions or variables. In this example, we check to see if the script is being run on a Friday.
if [ `date +”%A”` == Friday ];then
echo “TGIF!”;
fi
The basic syntax for an if command is “if value == other_value”. The == performs a comparison, and it’s necessary to ensure that the shell sees one value on each side of the comparison operator. For this reason, you often have to put your strings in quotes of some kind.
Understanding variables
Just to be sure this is clear, it’s important to understand that variables are assigned in one way and referenced in another. Assign a variable with just its name, but precede the name with a $ to use it.
$ favnum=06
$ echo $favnum
06
Prompting the user for input
To prompt a user within a script to enter some information, you need to provide both the prompt and the command to read what the user types. You should also assign a variable name that makes sense as in this example. Note that using the echo -n command means the user will enter their response on the same line as the prompt is displayed.
echo -n “How many loops?> “
read numloops
The person running the script will see the prompt and provide their response:
How many loops?> 11
Using command line arguments
To make use of arguments that a user enters along with the script name, you need to know how to identify them. Script arguments will be assigned the names $1, $2 and so on. For any argument that you’re going to use repeatedly, you might consider assigning those values to more meaningful variable names.
$!/bin/bash
if [[ $1 != [0-9]* ]]; then
echo “Error: $1 is not numeric”
exit
else
loops=$1
fi
In this case, we’re checking to be sure that the first argument provided is numeric and exiting the script if it is not. If the response is numeric, we then assign it to the variable $loops to use later in the script.
Another useful thing to do in a script is to first verify that there are arguments. Otherwise syntax like what is shown above would fail because the shell would see “if [[ != [0-9]* ]];” which would generate a syntactical error.
To check that the number of arguments provided is correct, you could use syntax like the following that checks to be sure at least two arguments have been provided and, otherwise, reminds the user that both a number of lines and file name are required:
#!/bin/bash
if [ $# -lt 2 ]; then
echo “Usage: $0 lines filename”
exit 1
fi
Different ways to loop
There are a number of ways to loop within a script. Use for when you want to loop a preset number of times. For example:
#!/bin/bash
for day in Sun Mon Tue Wed Thu Fri Sat
do
echo $day
done
OR
#!/bin/bash
for letter in {a..z}
do
echo $letter
done
Use while when you want to loop as long as some condition exists or doesn’t exist.
#!/bin/bash
n=1
while [ $n -le 4 ]
do
echo $n
((n++))
done
Using case statements
Case statements allow your scripts to react differently depending on what values are being examined. In the script below, we use different commands to extract the contents of the file provided as an argument by identifying the file type.
#!/bin/bash
if [ $# -eq 0 ]; then
echo -n “filename> “
read filename
else
filename=$1
fi
if [ ! -f “$filename” ]; then
echo “No such file: $filename”
exit
fi
case $filename in
*.tar) tar xf $filename;;
*.tar.bz2) tar xjf $filename;;
*.tbz) tar xjf $filename;;
*.tbz2) tar xjf $filename;;
*.tgz) tar xzf $filename;;
*.tar.gz) tar xzf $filename;;
*.gz) gunzip $filename;;
*.bz2) bunzip2 $filename;;
*.zip) unzip $filename;;
*.Z) uncompress $filename;;
*.rar) rar x $filename ;;
*) echo “No extract option for $filename”
esac
Note that this script also prompts for a file name if none was provided and then checks to make sure that the file specified actually exists. Only after that does it bother with the extraction.
Reacting to errors
You can detect and react to errors within scripts and, in doing so, avoid other errors. The trick is to check the exit codes after commands are run. If an exit code has a value other than zero, an error occurred.
In this script, we look to see if Apache is running, but send the output from the check to /dev/null. We then check to see if the exit code isn’t equal to zero as this would indicate that the ps command did not get a response. If the exit code is not zero, the script informs the user that Apache isn’t running.
#!/bin/bash
# Script Name : CheckApache
# Script Info: Check Apache services
# Created by/On : ImJhaChandan/29-08-2021
ps -ef | grep apache2 > /dev/null
if [ $? != 0 ]; then
echo Apache is not running
exit
fi
In the end conclusion is that Scripts on Linux/Unix systems can help ensure that complicated processing is done in a consistent manner and makes running a series of commands a lot less trouble.
That's all in this post. If you liked this blog and interested in knowing more about UNIX / LINUX / AIX. Please Like, Follow, Share & Subscribe to www.ImJhaChandan.com
Commentaires